Iterations
The iteration tag allows code to be executed repeatedly.
In the section Input we saw that you can make your own custom variables and store data in each object. You can also store collections (group of data) in a custom drop. This can be done by iterating over the namespace and then using the custom drop as a dictionary.
Fori loop
In this example, we like to make a database (collection) that is called custom.the_namespace.
In this collection, we create attributes (variables) into our collection : some_data.
Because of the for loop, the input code will be executed repeatedly (eg. every time you put in new data).
To make a link between the collection and the attributes, we use the_key : item.
We now made our custom collection, with multiple variables in it that are called:
custom.the_namespace.the_namespace_1.some_data
custom.the_namespace.the_namespace_2.some_data
custom.the_namespace.the_namespace_3.some_data
...
{% fori item in custom.the_namespace %}
{% input item.some_data %}
{% endfori %}
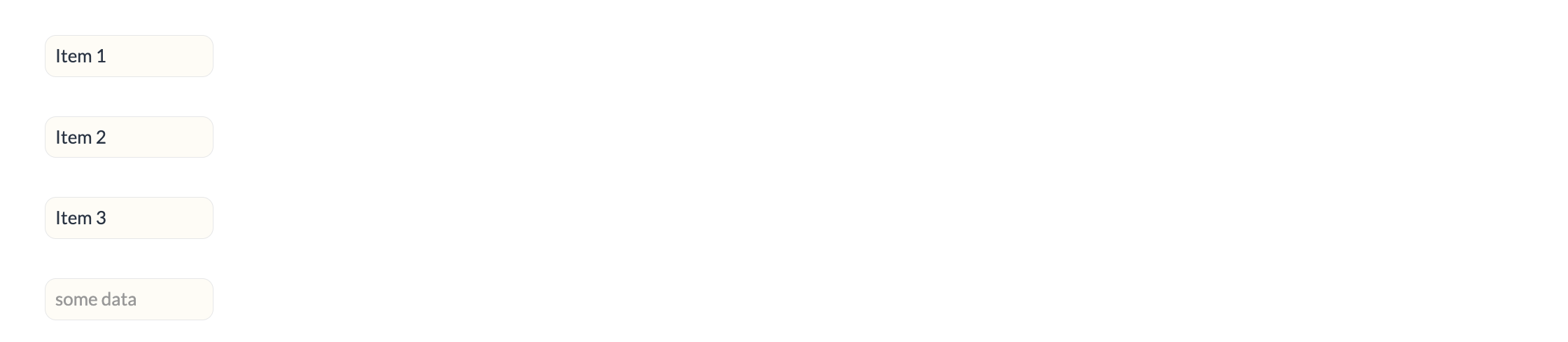
Inputs in a fori (custom collection) can be filled out by uploading data using the "Import reconciliation data" action on the template.
Import_title
When there is more than one custom collection with a fori in your template, you can add the import_title
attribute to distinguish between the collections. When using the "Import reconciliation data" action, users need to first choose to which collection they wish to upload data. By default we display the namespace of the collection.
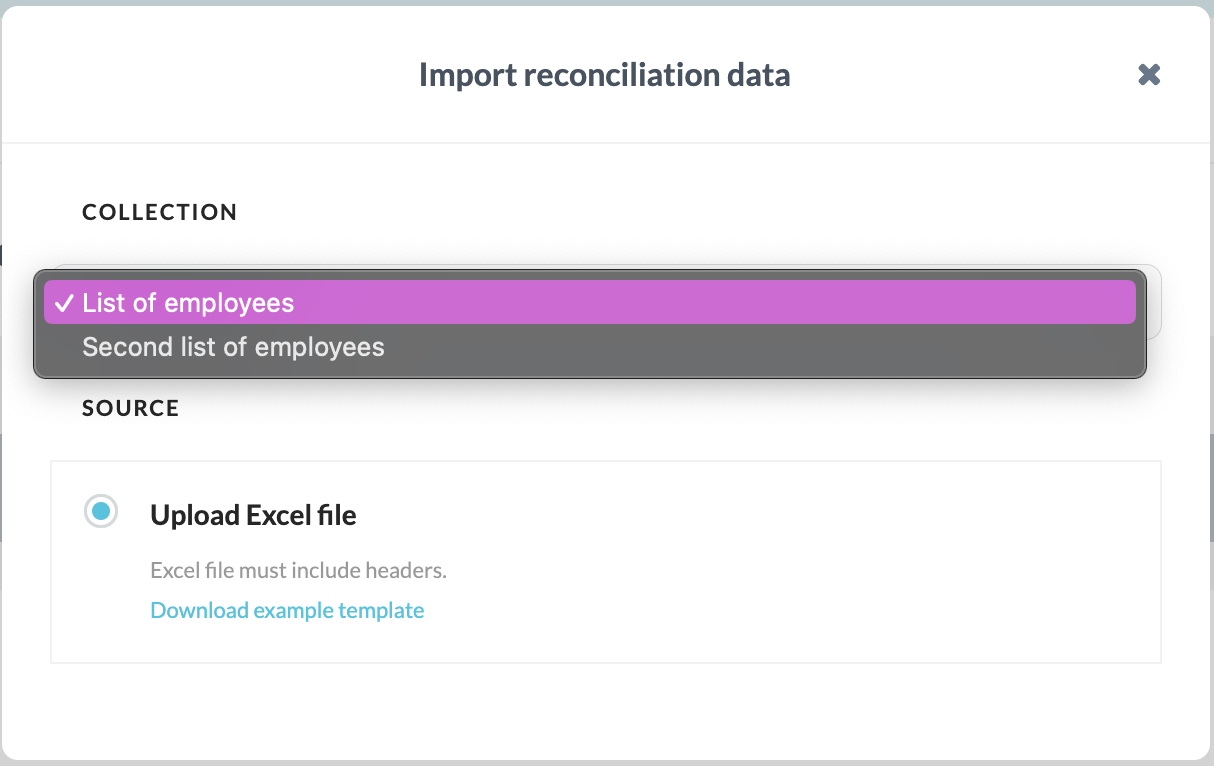
{% fori employee in custom.employees import_title:"List of employees" %}
{% input employee.name %}
{% input employee.date_of_birth as:date placeholder:'dd/mm/yyyy' import_title:'Date of birth' %}
{% endfori %}
{% fori employee in custom.employees2 import_title:"Second list of employees" %}
{% input employee.name %}
{% input employee.date_of_birth as:date placeholder:'dd/mm/yyyy' import_title:'Date of birth' %}
{% endfori %}
The Import_title attribute can also be used on input fields within a fori.
For loop
To print all the variables in our custom collection, we can print all variables individually:
{{ custom.the_namespace.the_namespace_1.some_data }}
{{ custom.the_namespace.the_namespace_2.some_data }}
{{ custom.the_namespace.the_namespace_3.some_data }}
...
Or we can use a for loop.
For loops can be used to iterate over collections Drops, a range of Numbers or Arrays.
{% for item in custom.the_namespace %}
{{ item.some_data}}
{% endfor %}
Example
Loop over an existing collection : collection Account will give all the accounts in the collection account that start with 611.
{% for account in period.accounts.611 %}
{{ account.number }}
{% endfor %}
611030
611060
Range
Loop through a range of numbers. A range definition looks like (1..10), defining between parentheses the start and end values for the range.
{% for i in (1..5) %}
{{ i }}
{% endfor %}
2
3
4
5
Helper variables
During the execution of every loop, you can use any of the following helpers.
For the examples below, assume we're using the same input every time, but each prints the relevant helper.
{% for i in (1..3) %}
{{ forloop.index }}
{% endfor %}
forloop.index
Return the index of the current iteration
1
2
3
forloop.index0
Return the index of the current iteration (zero based)
0
1
2
forloop.rindex
Return the index of the current iteration in descending order
3
2
1
forloop.length
Return, in each iteration, the length of the entire loop.
3
3
3
forloop.first
Return a boolean checking if this is the first loop.
true
false
false
forloop.last
Return a boolean checking if this is the last loop.
false
false
true
Iteration tags
break
Causes the loop to stop iterating when it encounters the break tag.
{% assign numbers = "1;2;3;4;5" | split:";" %}
{% for item in numbers %}
{{ item }}
{% if INT(item) > 2 %}
{% break %}
{% endif %}
{% endfor %}
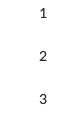
continue
Causes the loop to skip the current iteration when it encounters the continue tag.
{% assign numbers = "1;2;3;4" | split:";" %}
{% for item in numbers %}
{% if INT(item) == 2 %}
{% continue %}
{% else %}
{{ item }}
{% endif %}
{% endfor %}
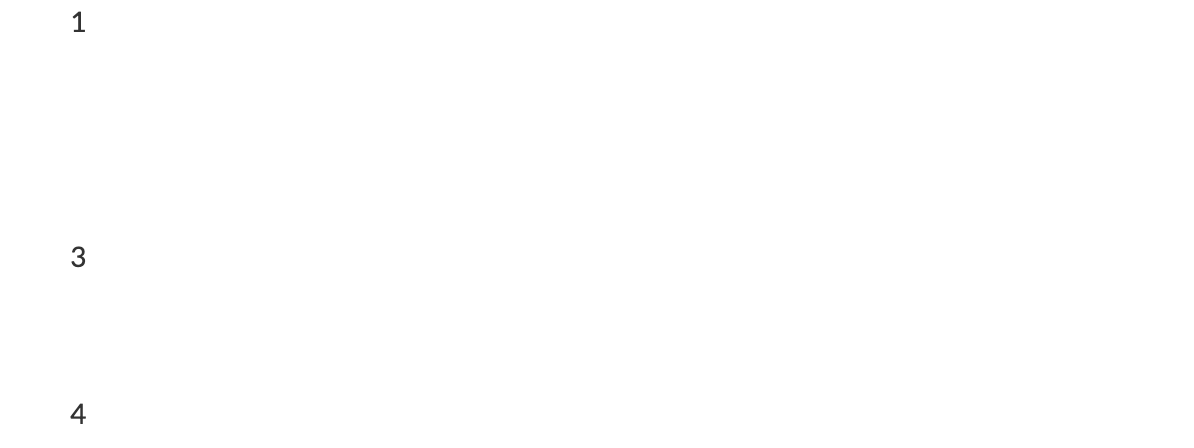
limit
The limit tag allows to exit the for loop at a specific index.
{% input custom.numberOfMonths.perPeriod as:select options:"1|2|3|4|5|6|7|8|9|10|11|12" default:"12" %}
{% assign numberOfMonths = custom.numberOfMonths.perPeriod | default:12 %}
{% for month in period.month_end_dates limit:INT(numberOfMonths) %}
**_{{ month | date:'%m/%Y'}}_**
{% endfor %}
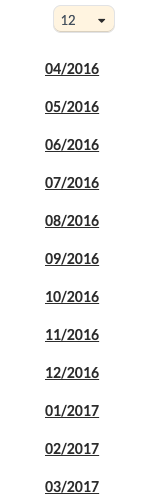
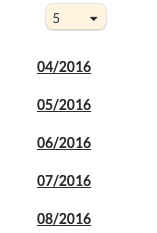
offset
Offset tag allows to start the for loop at a specific index.
{% assign numbers = "1;2;3;4;5" | split:";" %}
{% for item in numbers offset:2 %}
{{ item }}
{% endfor %}
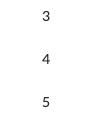
reversed
Reversed tag allows to reverse the order of the loop.
{% assign numbers = "1;2;3;4;5" | split:";" %}
{% for item in numbers reversed %}
{{ item }}
{% endfor %}
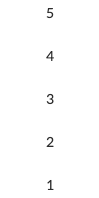
Updated 6 months ago