Operators
Comparing variables.
Operators are used to compare values.
In combination with control flow tags, you can put some logic in your code. Control flow tags create conditions that decide whether blocks of code get executed.
To explain all operators, we will be using the control flow: if statement and the tag: assign.
Content
Name | Use | Symbol |
---|---|---|
Equal | Check if two values are the same | == |
Difference | Check if two values are different | != |
Greater than | Check if the value on the left side is larger than the value on the right side | > |
Greater than or equal | Check if the value on the left side is larger or equal than the value on the right side | >= |
Smaller than | Check if the value on the right side is larger than the value on the left side | < |
Smaller than or equal | Check if the value on the right side is larger or equal than the value on the left side | <= |
Or | Combine two or more of the above operators. | or |
And | Combine two or more of the above operators | and |
Contains | Check if a given string is part of a another string | contains |
A String is a trail of successive characters. The string stands between quotation marks "string", to indicate where the trail of characters begins and ends.
Equal
The equal operator ==
checks if two values are the same. These can be numbers, as well as strings.
{% assign var1 = 100 %}
{% assign var2 = 150 %}
{% if var1 == var2 %}
The values are the same
{% else %}
The values are different
{% endif %}
Difference
The difference operator !=
checks if two values are different from each other. These can be numbers as well as strings.
{% assign var1 = 100 %}
{% assign var2 = 150 %}
{% if var1 != var2 %}
The values are different
{% else %}
The values are the same
{% endif %}
Greater than
The greater than operator >
checks if the value on the left side of the operator is larger than the value on the right side of the operator.
{% assign var1 = 100 %}
{% assign var2 = 150 %}
{% if var1 > var2 %}
{{ var1 }} is larger than {{ var2 }}
{% else %}
{{ var1 }} is smaller than {{ var2 }}
{% endif %}
Greater than or equal
The greater than operator >=
checks if the value on the left side of the operator is larger than or equal to the value on the right side of the operator.
{% assign var1 = 100 %}
{% assign var2 = 150 %}
{% if var1 >= var2 %}
{{ var1 }} is larger than or equal to {{ var2 }}
{% else %}
{{ var1 }} is smaller than {{ var2 }}
{% endif %}
Smaller than
The smaller than operator <
checks if the left value on the left side of the operator is smaller than the value on the right side of the operator.
{% assign var1 = 100 %}
{% assign var2 = 150 %}
{% if var1 < var2 %}
{{ var1 }} is smaller than {{ var2 }}
{% else %}
{{ var1 }} is larger than {{ var2 }}
{% endif %}
Smaller than or equal
The smaller than operator or equal to operator <=
checks if the left value on the left side of the operator is smaller than or equal to the value on the right side of the operator.
{% assign var1 = 100 %}
{% assign var2 = 150 %}
{% if var1 <= var2 %}
{{ var1 }} is larger than or equal to {{ var2 }}
{% else %}
{{ var1 }} is larger than {{ var2 }}
{% endif %}
Or
The operator or
makes it possible to combine two or more of the above operators. If one of the statements is true, the code will be executed.
The or operator is case sensitive.
{% assign var1 = 100 %}
{% assign var2 = 150 %}
{% if var1 > 0 or var2 > 0 %}
One of the variables is larger than 0
{% else %}
Both variables are smaller than 0
{% endif %}
And
The operator and
makes it possible to combine two or more of the above operators. If one of the statements is true, the code will be executed.
The and operator is case sensitive.
{% assign var1 = 100 %}
{% assign var2 = 150 %}
{% if var1 > 0 and var2 > 0 %}
Both variables are larger than 0
{% else %}
At least one of the variables is smaller than 0
{% endif %}
In tags with more than one and or or operator, operators are checked in order from right to left. You cannot change the order of operations using parentheses — parentheses are invalid characters in Silverfin template language and will prevent your tags from working.
Contains
Strings
The contains
operator checks if a given string (the needle) is part of another string (the stack).
In this example we search for the string "profit" (the needle) in another string "The profit of the current year is 1.000,00" (the stack).
The contains operator is case sensitive.
{% assign sentence = "The profit of the current year is 1.000,00" %}
{% if sentence contains "profit" %}
The sentence *{{sentence}}* contains the word profit.
{% else %}
The sentence *{{sentence}}* does not contain the word profit.
{% endif %}
Account Collections
Apart from strings, the contains
operator can be used to check if a given account is part of the collection. For example:
{% assign costs = period.accounts | range:6 %}
{% input custom.some.accounts as:account_collection range:"6,7" accounts_var:some_accounts %}
{% for account in some_accounts %}
{% if costs contains account %} {{ account.number }} is part of the 'Costs' collection{% endif %}
{% endfor %}
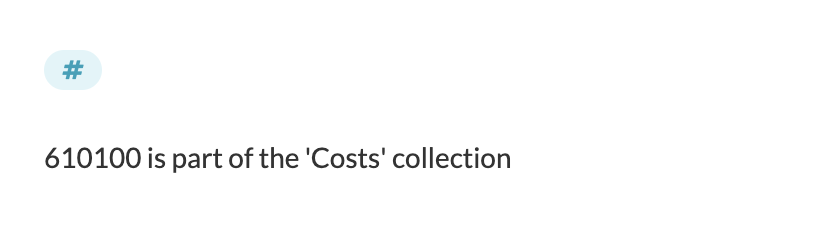
Updated about 2 years ago